optimistic captcha?
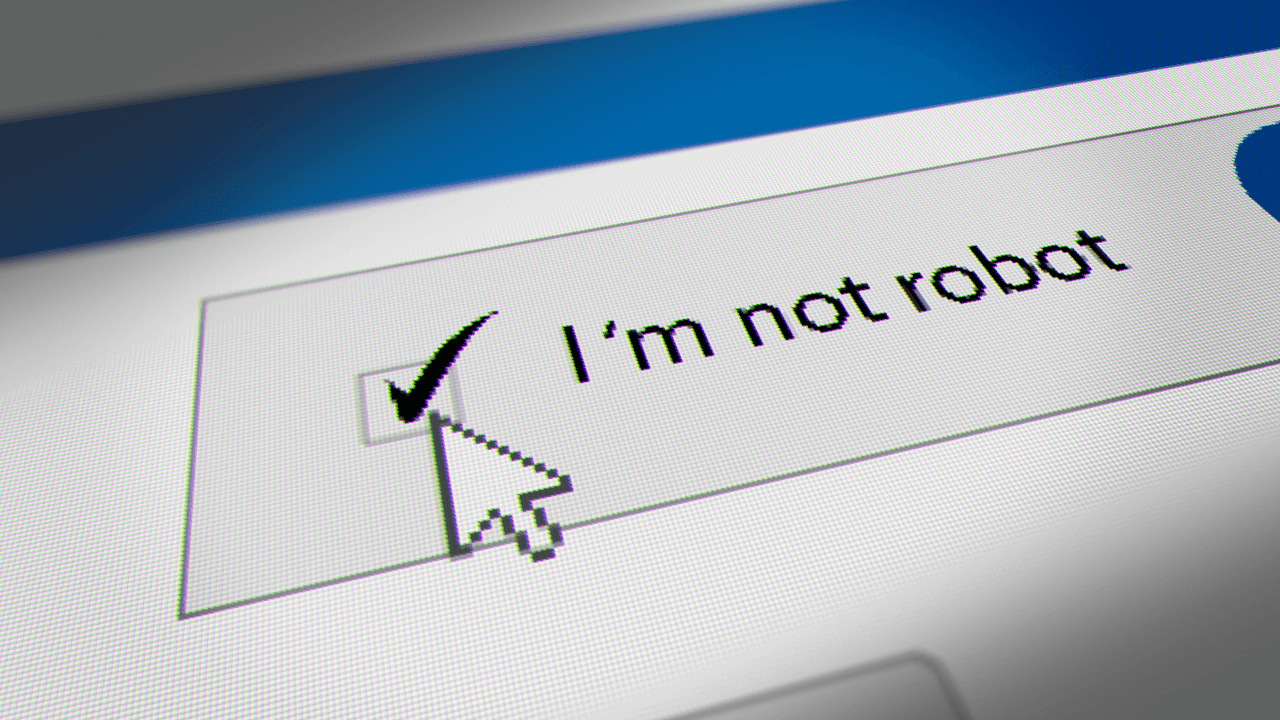
so, you want to use google recaptcha (or other rest validaton based captchas) but don’t want to wait 2-3 sec’s to get response?
what if?
we assume that captcha will be successful and process the action which user requested and revert (or revoke) this action only if when provided captcha is not valid?
with this way, regular users won’t suffer slow response times and we may still be able to prevent unwanted access.
async function verifyOTP ({ request }) {
const { phoneNumber, otpCode, captcha } = request.body;
// check db for phoneNumber, otpCode
if (!isValid(phoneNumber, otpCode)) {
// consume captcha synchronously and give response accordingly
// so attackers can't use and gain intel with wrong captchas
const captchaOK = await VerifyCaptcha({ captcha })
if (captchaOK) {
throw new Error('otp not valid')
}
throw new Error('invalid captcha')
}
const user = getUserByPhoneNumber(phoneNumber)
const accessToken = generateAccessToken(user)
// now we trigger a job (or background function) for checking captcha
// and if it's not valid, we will revoke access token
jobs.trigger('VerifyCaptcha', { accessToken, captcha })
return user
}
async function VerifyCaptcha ({ accessToken, captcha }) {
// this may take 2-3 seconds
const isCaptchaValid = await CHECK_CAPTCHA_FROM_VENDOR(captcha)
if (isCaptchaValid) {
return true
}
if (accessToken) {
// revoke accessToken somehow
await Tokens.delete(accessToken)
}
return false
}
warning
please think twice while you are implementing this, because this approach may create security vulnerabilities.
for example if you secure your application’s login endpoint
with optimistic captcha; even if you revoke their tokens on attemps with failed captchas; you are giving bruteforce ability to attackers
.
i saw first implementation on this while researching some mobile application's api.
but because of the obvious reasons, i'm not able to share it